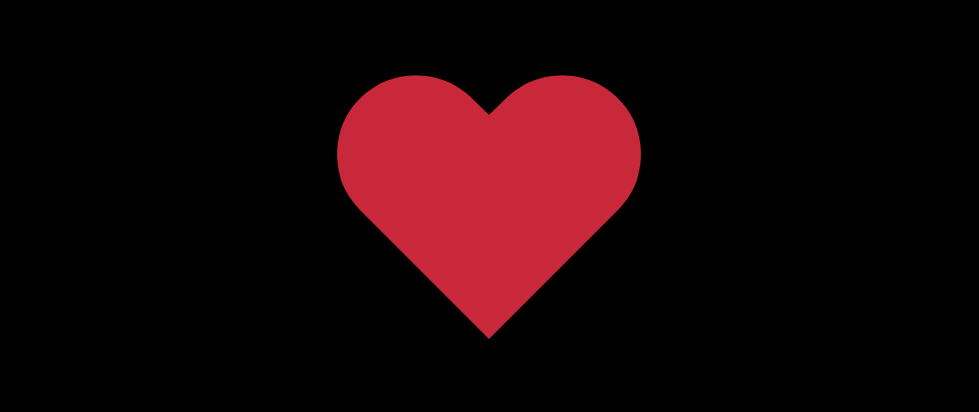
Tiny Toolkit - how to go big
Today we’re going meta and doing a Tiny Tutorial™ on creating a Tiny Toolkit™ to use in our future Tiny Tutorials. We’re creating some code that will take a content div full-screen and back on desktop and mobile devices.
Lets create a canvas for our work of digital art, in a stylish and hip anamorphic aspect ratio using some decidedly un-hip super-old-school CSS padding techniques.
.display-container {
width: 100%;
}
.display-aspect {
width: 100%;
padding-top: 41.841%; /* -- 21:9 aspect ratio - our demos are anamorphic! -- */
position: relative;
}
.display {
background-color: black;
position: absolute;
top: 0;
left: 0;
right: 0;
bottom: 0;
}
Which applied to this nested russian doll of divs will make the display div height proportional to its width.
<div class="display-container">
<div class="display-aspect">
<div class="display" id="display">
future content
</div>
</div>
</div>
Start listening to keypress events, but ignore everything that’s not the Enter key or the f(ullscreen) key.
document.addEventListener(
'keydown',
(e) => {
if (e.key === 'Enter' || e.key.toLowerCase() === 'f') {
toggleFullScreen()
}
},
false,
)
But how are we going to press keys on touch devices? Good point, let’s hook double tap as well. Returning false stops the default double-click behaviour, which on most touch devices is an unwanted screen zoom.
document.getElementById('display').addEventListener('click', displayTapped, false)
function displayTapped(event) {
if (event.detail === 2) {
toggleFullScreen()
return false
}
}
Oops better do something when we trigger these events. Look how clean and elegant this is, web standards for the win.
function toggleFullScreen() {
if (document.fullscreenElement) {
document.exitFullscreen()
} else {
document.documentElement.requestFullscreen()
}
}
Safari you rascally scamp - did you implement a standard with non-standard names? You sure did! Now my code is as ugly as your implementation, so we're both losers.
function toggleFullScreen() {
+ if (document.fullscreenElement || document.webkitFullscreenElement) {
+ if (document.webkitExitFullscreen) {
+ document.webkitExitFullscreen()
+ } else {
document.exitFullscreen()
+ }
} else {
+ if (document.documentElement.webkitRequestFullscreen) {
+ document.documentElement.webkitRequestFullscreen()
+ } else {
document.documentElement.requestFullscreen()
+ }
}
}
Add a class that makes the display content expand to fill the entire screen.
.display-full-screen {
position: fixed !important;
z-index: 10000;
}
Now lets listen for the full screen change event. When we go full screen we add the display-full-screen class to our display element so it covers the whole screen. We’ll also disable scrolling so those pesky scrollbars go away.
Conversely when we go back to normal view, we remove the CSS class and re-enable scrolling.
It’s important to capture the fullscreenchange event instead of doing these actions in toggleFullScreeen() as the user could press escape or use the menu to go out of full screen mode. This approach ensures everything behaves as they expect and they don’t freak out.
document.addEventListener('fullscreenchange', screenChangeHandler, false)
function screenChangeHandler() {
if (document.fullscreenElement) {
document.getElementById('display').classList.add('display-full-screen')
document.body.style.overflow = 'hidden'
} else {
document.getElementById('display').classList.remove('display-full-screen')
document.body.style.overflow = 'auto'
}
}
Damn you Safari.
document.addEventListener('fullscreenchange', screenChangeHandler, false)
+document.addEventListener('webkitfullscreenchange', screenChangeHandler, false)
function screenChangeHandler() {
+ if (document.fullscreenElement || document.webkitFullscreenElement) {
document.getElementById('display').classList.add('display-full-screen')
document.body.style.overflow = 'hidden'
} else {
document.getElementById('display').classList.remove('display-full-screen')
document.body.style.overflow = 'auto'
}
}
It’s all working but double clicking causes content in our display div to highlight. A quick bit of CSS will put an end to that ugliness.
.display {
user-select: none;
}
Of course.
.display {
+ -webkit-user-select: none; // Safari
user-select: none; // Every other browser in the world
}
When web developers say mean things about Safari being the new Internet Explorer 6, this is what they’re talking about.
Conclusion
Now we have the start of our tiny DemoKit™ toolkit. We can double tap or press the Enter key or F key to toggle between full screen and normal.
Of course size is important, and BundlePhobia says DemoKit™ is only 0.47kb!
Just kidding, I’m not going to publish this and neither should you. It’s barely more useful than leftPad and we all know how that turned out.
If you want to see the final code, view the source of this page.